Dream Map Ting
Monday, November 8, 2010
Week13 – Final report and research project
Hey,
This is the link for my final report. Click here
This is the link for my research project. Click here
Friday, October 29, 2010
Week12 – PHP and MYSQL LOGIN part
I think the following code might be help to who also has login function on the website.
Step1:
create table on your database.
create table tab_admin(
id int(10) auto_increment primary key,
loginame varchar(10) not null,
password varchar(12) not null
)
insert into tab_admin values (' ','adminname','adminpassword');
Step2:
create form on the web page
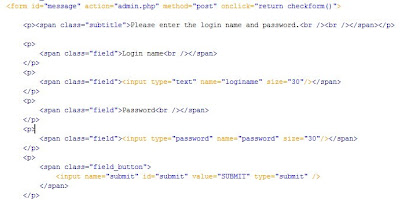
create new .php page
?php
$user="******";
$password="**********";
$database="******";
$link = mysql_connect("rerun.it.uts.edu.au", $user, $password) or die("Unable to connect to database");
$db = mysql_select_db($database, $link) or die(mysql_error());$host="localhost"; // Host name
$tbl_name="tab_admin"; // Table name
$loginame=$_POST['loginame'];
$password=$_POST['password'];
// To protect MySQL injection (more detail about MySQL injection)
$loginame = stripslashes($loginame);
$password = stripslashes($password);
$loginame = mysql_real_escape_string($loginame);
$password = mysql_real_escape_string($password);
$sql="SELECT * FROM $tbl_name WHERE loginame='$loginame' and password='$password'";
$result=mysql_query($sql);
// Mysql_num_row is counting table row
$count=mysql_num_rows($result);
// If result matched $myusername and $mypassword, table row must be 1 row
if($count==1){
// Register $myusername, $mypassword and redirect to file "login_success.php"
session_register("loginame");
session_register("password");
header("location:login_success.php");
}
else {
echo 'Wrong Username or Password';
}
?>
Step4:
create login_successful.php
session_start();
session_destroy();
?>
DONE~~~~Finish the login function
Reference: http://php.about.com/od/finishedphp1/ss/php_login_code.htm
Sunday, October 24, 2010
Week11 – Working on the web system
I found some useful website about the PHP and MySQL tutorial.
This is the most clear one to introduce how to use PHP and connect with MySQL in your project from very beginning. http://www.freewebmasterhelp.com/tutorials/phpmysql
Also there are some tips for HTML button 'onclick' event summary.
input onclick="document.all.WebBrowser.ExecWB(1,1)" value="OPEN" name="Button1" type="button"
input onclick="document.all.WebBrowser.ExecWB(4,1)" value="SAVE AS" name="Button2" type="button"
input onclick="document.all.WebBrowser.ExecWB(6,1)" value="print" name="Button" type="button"
input onclick="window.location.reload()" value="refresh" name="refresh" type="button"
input onclick="window.external.AddFavorite(location.href, document.title)" value="add to my favorite" name="Button22" type="button"
input onclick="'window.location=" value="check page sources" name="Button7" type="button"
input onclick="document.execCommand('Cut')" value="cut" type="button"
input onclick="document.execCommand('Copy')" value="copy" type="button"
input onclick="document.execCommand('Paste')" value="paste" type="button"
input onclick="document.execCommand('Undo')" value="undo" type="button"
input onclick="document.execCommand('Delete')" value="delete" type="button"
input onclick="document.execCommand('Bold')" value="Bold" type="button"
input onclick="document.execCommand('Italic')" value="Italic" type="button"
input onclick="document.execCommand('Underline')" value="Underline" type="button"
input onclick="document.execCommand('stop')" value="stop" type="button"
input onclick="document.execCommand('SaveAs')" value="Save" type="button"
input onclick="document.execCommand('Saveas',false,'c:\\Autorun.inf')" value="Save as" type="button"
input onclick="document.execCommand('FontName',false,fn)" value="Font" type="button"
input onclick="document.execCommand('FontSize',false,fs)" value="FontSize" type="button"
input onclick="document.execCommand('refresh',false,0)" value="refresh" type="button"
input onclick="window.location.reload()" value="refresh" type="button"
input onclick="history.go(1)" value="go forward one page" type="button"
input onclick="history.go(-1)" value="back one page" type="button"
input onclick="history.forward()" value="go forward" type="button"
input onclick="history.back()" value="back" type="button"
input value="open new window" onclick="javascript:window.open('#','','scrollbars=yes,width=600,height=200')" type="button"
input value="link to page" onclick="window.location.href=''" type="button"
input value="previous page" onclick="javascript:history.go(-1);" type="button"
Tuesday, October 19, 2010
Week10 – Database design
According to my final project system plan, I created my database. There are sixes tables. The following diagram shows the relationships between each other.

Not only listing the relationship, but also listing the primary key and foreign key among these tables. Table tab_restaurant, tab_menu, tab_type and tab_image are used to store the information about restaurant. Table tab_order is used to record the customer's order and its status. Table tab_message is to record leaving message from customer. I'm still thinking about create the administrator account in order to better manage the web system.
Monday, October 11, 2010
Monday, October 4, 2010
Week9 – SQL practice in Class
Q1: Use the INSERT command to create a couple of new books in the table
INSERT INTO `tliu`.`books` (`bookid`, `title`, `Author`, `ISBN`, `cost`) VALUES (NULL , 'Thinking in JAVA', 'Andy', '234123567', '18');
INSERT INTO `tliu`.`books` (`bookid`, `title`, `Author`, `ISBN`, `cost`) VALUES (NULL, 'C# introduction', 'Willey', '456743257532', '9');
Q2: Use the SELECT command to find all books whose title begins with W
SELECT *
FROM `books`
WHERE title LIKE 'W%'
Q3: Use the SELECT command to find all books whose price is less than 10
SELECT *
FROM `books`
WHERE cost < '10'
Q4: Use a LIMIT clause on a SELECT command to just list the first two books
SELECT *
FROM `books`
LIMIT 2
Q5: Use both LIMIT and ORDER BY in a SELECT command
SELECT *
FROM `books`
ORDER BY bookid
LIMIT 2
Comments: I found the Q4 and Q5 are the same answer.
Thursday, September 23, 2010
Week8 – Javascrpit Prac in Class
I'm doing this week practice about JavaScript. I finished Challenge Exercise, but I got the bug on the basic one. Who can help me?
Firstly, click here.
When you open the page, it should pop up the current date by coding:
var today = new Date();
alert(('today is ') + today);
And then, you can find the button named 'how many days old I am' on the bottom of the page. Click the button, and the days will display by coding:
var today = new Date();
(This is current date and time.)
var my_date = "1985,10,25 04:00:00";
(This is my birthday and time.)
var time1 = new Date(my_date);
var time2 = new Date(today);
var days = (time2.getTime() - time1.getTime())/86400000;
input value="how many days old I am" onclick="alert((days)+' days')" type="button"
However, the days is float. Question one: how to display only the integrate number?
Another bug is click the small pic, the following pic can't change except the first one. I don't know why. I try to open this page on my local computer, it works. But when I upload the folder into FTP client, it does not work. Who can help me?
Cheers,
Tingting